- Data Types in Go
- Go Keywords
- Go Control Flow
- Go Functions
- GoLang Structures
- GoLang Arrays
- GoLang Strings
- GoLang Pointers
- GoLang Interface
- GoLang Concurrency

strconv.Atoi() Function in Golang With Examples
Go language provides inbuilt support to implement conversions to and from string representations of basic data types by strconv Package. This package provides an Atoi() function which is equivalent to ParseInt(str string, base int, bitSize int) is used to convert string type into int type. To access Atoi() function you need to import strconv Package in your program.
Here, str is the string.
Please Login to comment...
Similar reads.
- Golang-strconv
- Go Language
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
This package is not in the latest version of its module.
Documentation ¶
- Numeric Conversions
- String Conversions
Package strconv implements conversions to and from string representations of basic data types.
Numeric Conversions ¶
The most common numeric conversions are Atoi (string to int) and Itoa (int to string).
These assume decimal and the Go int type.
ParseBool , ParseFloat , ParseInt , and ParseUint convert strings to values:
The parse functions return the widest type (float64, int64, and uint64), but if the size argument specifies a narrower width the result can be converted to that narrower type without data loss:
FormatBool , FormatFloat , FormatInt , and FormatUint convert values to strings:
AppendBool , AppendFloat , AppendInt , and AppendUint are similar but append the formatted value to a destination slice.
String Conversions ¶
Quote and QuoteToASCII convert strings to quoted Go string literals. The latter guarantees that the result is an ASCII string, by escaping any non-ASCII Unicode with \u:
QuoteRune and QuoteRuneToASCII are similar but accept runes and return quoted Go rune literals.
Unquote and UnquoteChar unquote Go string and rune literals.
- func AppendBool(dst []byte, b bool) []byte
- func AppendFloat(dst []byte, f float64, fmt byte, prec, bitSize int) []byte
- func AppendInt(dst []byte, i int64, base int) []byte
- func AppendQuote(dst []byte, s string) []byte
- func AppendQuoteRune(dst []byte, r rune) []byte
- func AppendQuoteRuneToASCII(dst []byte, r rune) []byte
- func AppendQuoteRuneToGraphic(dst []byte, r rune) []byte
- func AppendQuoteToASCII(dst []byte, s string) []byte
- func AppendQuoteToGraphic(dst []byte, s string) []byte
- func AppendUint(dst []byte, i uint64, base int) []byte
- func Atoi(s string) (int, error)
- func CanBackquote(s string) bool
- func FormatBool(b bool) string
- func FormatComplex(c complex128, fmt byte, prec, bitSize int) string
- func FormatFloat(f float64, fmt byte, prec, bitSize int) string
- func FormatInt(i int64, base int) string
- func FormatUint(i uint64, base int) string
- func IsGraphic(r rune) bool
- func IsPrint(r rune) bool
- func Itoa(i int) string
- func ParseBool(str string) (bool, error)
- func ParseComplex(s string, bitSize int) (complex128, error)
- func ParseFloat(s string, bitSize int) (float64, error)
- func ParseInt(s string, base int, bitSize int) (i int64, err error)
- func ParseUint(s string, base int, bitSize int) (uint64, error)
- func Quote(s string) string
- func QuoteRune(r rune) string
- func QuoteRuneToASCII(r rune) string
- func QuoteRuneToGraphic(r rune) string
- func QuoteToASCII(s string) string
- func QuoteToGraphic(s string) string
- func QuotedPrefix(s string) (string, error)
- func Unquote(s string) (string, error)
- func UnquoteChar(s string, quote byte) (value rune, multibyte bool, tail string, err error)
- type NumError
- func (e *NumError) Error() string
- func (e *NumError) Unwrap() error
- AppendFloat
- AppendQuote
- AppendQuoteRune
- AppendQuoteRuneToASCII
- AppendQuoteToASCII
- CanBackquote
- FormatFloat
- QuoteRuneToASCII
- QuoteRuneToGraphic
- QuoteToASCII
- QuoteToGraphic
- QuotedPrefix
- UnquoteChar
Constants ¶
IntSize is the size in bits of an int or uint value.
Variables ¶
ErrRange indicates that a value is out of range for the target type.
ErrSyntax indicates that a value does not have the right syntax for the target type.
Functions ¶
Func appendbool ¶.
AppendBool appends "true" or "false", according to the value of b, to dst and returns the extended buffer.
func AppendFloat ¶
AppendFloat appends the string form of the floating-point number f, as generated by FormatFloat, to dst and returns the extended buffer.
func AppendInt ¶
AppendInt appends the string form of the integer i, as generated by FormatInt, to dst and returns the extended buffer.
func AppendQuote ¶
AppendQuote appends a double-quoted Go string literal representing s, as generated by Quote, to dst and returns the extended buffer.
func AppendQuoteRune ¶
AppendQuoteRune appends a single-quoted Go character literal representing the rune, as generated by QuoteRune, to dst and returns the extended buffer.
func AppendQuoteRuneToASCII ¶
AppendQuoteRuneToASCII appends a single-quoted Go character literal representing the rune, as generated by QuoteRuneToASCII, to dst and returns the extended buffer.
func AppendQuoteRuneToGraphic ¶ added in go1.6
AppendQuoteRuneToGraphic appends a single-quoted Go character literal representing the rune, as generated by QuoteRuneToGraphic, to dst and returns the extended buffer.
func AppendQuoteToASCII ¶
AppendQuoteToASCII appends a double-quoted Go string literal representing s, as generated by QuoteToASCII, to dst and returns the extended buffer.
func AppendQuoteToGraphic ¶ added in go1.6
AppendQuoteToGraphic appends a double-quoted Go string literal representing s, as generated by QuoteToGraphic, to dst and returns the extended buffer.
func AppendUint ¶
AppendUint appends the string form of the unsigned integer i, as generated by FormatUint, to dst and returns the extended buffer.
func Atoi ¶
Atoi is equivalent to ParseInt(s, 10, 0), converted to type int.
func CanBackquote ¶
CanBackquote reports whether the string s can be represented unchanged as a single-line backquoted string without control characters other than tab.
func FormatBool ¶
FormatBool returns "true" or "false" according to the value of b.
func FormatComplex ¶ added in go1.15
FormatComplex converts the complex number c to a string of the form (a+bi) where a and b are the real and imaginary parts, formatted according to the format fmt and precision prec.
The format fmt and precision prec have the same meaning as in FormatFloat. It rounds the result assuming that the original was obtained from a complex value of bitSize bits, which must be 64 for complex64 and 128 for complex128.
func FormatFloat ¶
FormatFloat converts the floating-point number f to a string, according to the format fmt and precision prec. It rounds the result assuming that the original was obtained from a floating-point value of bitSize bits (32 for float32, 64 for float64).
The format fmt is one of 'b' (-ddddp±ddd, a binary exponent), 'e' (-d.dddde±dd, a decimal exponent), 'E' (-d.ddddE±dd, a decimal exponent), 'f' (-ddd.dddd, no exponent), 'g' ('e' for large exponents, 'f' otherwise), 'G' ('E' for large exponents, 'f' otherwise), 'x' (-0xd.ddddp±ddd, a hexadecimal fraction and binary exponent), or 'X' (-0Xd.ddddP±ddd, a hexadecimal fraction and binary exponent).
The precision prec controls the number of digits (excluding the exponent) printed by the 'e', 'E', 'f', 'g', 'G', 'x', and 'X' formats. For 'e', 'E', 'f', 'x', and 'X', it is the number of digits after the decimal point. For 'g' and 'G' it is the maximum number of significant digits (trailing zeros are removed). The special precision -1 uses the smallest number of digits necessary such that ParseFloat will return f exactly.
func FormatInt ¶
FormatInt returns the string representation of i in the given base, for 2 <= base <= 36. The result uses the lower-case letters 'a' to 'z' for digit values >= 10.
func FormatUint ¶
FormatUint returns the string representation of i in the given base, for 2 <= base <= 36. The result uses the lower-case letters 'a' to 'z' for digit values >= 10.
func IsGraphic ¶ added in go1.6
IsGraphic reports whether the rune is defined as a Graphic by Unicode. Such characters include letters, marks, numbers, punctuation, symbols, and spaces, from categories L, M, N, P, S, and Zs.
func IsPrint ¶
IsPrint reports whether the rune is defined as printable by Go, with the same definition as unicode.IsPrint: letters, numbers, punctuation, symbols and ASCII space.
func Itoa ¶
Itoa is equivalent to FormatInt(int64(i), 10).
func ParseBool ¶
ParseBool returns the boolean value represented by the string. It accepts 1, t, T, TRUE, true, True, 0, f, F, FALSE, false, False. Any other value returns an error.
func ParseComplex ¶ added in go1.15
ParseComplex converts the string s to a complex number with the precision specified by bitSize: 64 for complex64, or 128 for complex128. When bitSize=64, the result still has type complex128, but it will be convertible to complex64 without changing its value.
The number represented by s must be of the form N, Ni, or N±Ni, where N stands for a floating-point number as recognized by ParseFloat, and i is the imaginary component. If the second N is unsigned, a + sign is required between the two components as indicated by the ±. If the second N is NaN, only a + sign is accepted. The form may be parenthesized and cannot contain any spaces. The resulting complex number consists of the two components converted by ParseFloat.
The errors that ParseComplex returns have concrete type *NumError and include err.Num = s.
If s is not syntactically well-formed, ParseComplex returns err.Err = ErrSyntax.
If s is syntactically well-formed but either component is more than 1/2 ULP away from the largest floating point number of the given component's size, ParseComplex returns err.Err = ErrRange and c = ±Inf for the respective component.
func ParseFloat ¶
ParseFloat converts the string s to a floating-point number with the precision specified by bitSize: 32 for float32, or 64 for float64. When bitSize=32, the result still has type float64, but it will be convertible to float32 without changing its value.
ParseFloat accepts decimal and hexadecimal floating-point numbers as defined by the Go syntax for floating-point literals . If s is well-formed and near a valid floating-point number, ParseFloat returns the nearest floating-point number rounded using IEEE754 unbiased rounding. (Parsing a hexadecimal floating-point value only rounds when there are more bits in the hexadecimal representation than will fit in the mantissa.)
The errors that ParseFloat returns have concrete type *NumError and include err.Num = s.
If s is not syntactically well-formed, ParseFloat returns err.Err = ErrSyntax.
If s is syntactically well-formed but is more than 1/2 ULP away from the largest floating point number of the given size, ParseFloat returns f = ±Inf, err.Err = ErrRange.
ParseFloat recognizes the string "NaN", and the (possibly signed) strings "Inf" and "Infinity" as their respective special floating point values. It ignores case when matching.
func ParseInt ¶
ParseInt interprets a string s in the given base (0, 2 to 36) and bit size (0 to 64) and returns the corresponding value i.
The string may begin with a leading sign: "+" or "-".
If the base argument is 0, the true base is implied by the string's prefix following the sign (if present): 2 for "0b", 8 for "0" or "0o", 16 for "0x", and 10 otherwise. Also, for argument base 0 only, underscore characters are permitted as defined by the Go syntax for integer literals .
The bitSize argument specifies the integer type that the result must fit into. Bit sizes 0, 8, 16, 32, and 64 correspond to int, int8, int16, int32, and int64. If bitSize is below 0 or above 64, an error is returned.
The errors that ParseInt returns have concrete type *NumError and include err.Num = s. If s is empty or contains invalid digits, err.Err = ErrSyntax and the returned value is 0; if the value corresponding to s cannot be represented by a signed integer of the given size, err.Err = ErrRange and the returned value is the maximum magnitude integer of the appropriate bitSize and sign.
func ParseUint ¶
ParseUint is like ParseInt but for unsigned numbers.
A sign prefix is not permitted.
func Quote ¶
Quote returns a double-quoted Go string literal representing s. The returned string uses Go escape sequences (\t, \n, \xFF, \u0100) for control characters and non-printable characters as defined by IsPrint.
func QuoteRune ¶
QuoteRune returns a single-quoted Go character literal representing the rune. The returned string uses Go escape sequences (\t, \n, \xFF, \u0100) for control characters and non-printable characters as defined by IsPrint. If r is not a valid Unicode code point, it is interpreted as the Unicode replacement character U+FFFD.
func QuoteRuneToASCII ¶
QuoteRuneToASCII returns a single-quoted Go character literal representing the rune. The returned string uses Go escape sequences (\t, \n, \xFF, \u0100) for non-ASCII characters and non-printable characters as defined by IsPrint. If r is not a valid Unicode code point, it is interpreted as the Unicode replacement character U+FFFD.
func QuoteRuneToGraphic ¶ added in go1.6
QuoteRuneToGraphic returns a single-quoted Go character literal representing the rune. If the rune is not a Unicode graphic character, as defined by IsGraphic, the returned string will use a Go escape sequence (\t, \n, \xFF, \u0100). If r is not a valid Unicode code point, it is interpreted as the Unicode replacement character U+FFFD.
func QuoteToASCII ¶
QuoteToASCII returns a double-quoted Go string literal representing s. The returned string uses Go escape sequences (\t, \n, \xFF, \u0100) for non-ASCII characters and non-printable characters as defined by IsPrint.
func QuoteToGraphic ¶ added in go1.6
QuoteToGraphic returns a double-quoted Go string literal representing s. The returned string leaves Unicode graphic characters, as defined by IsGraphic, unchanged and uses Go escape sequences (\t, \n, \xFF, \u0100) for non-graphic characters.
func QuotedPrefix ¶ added in go1.17
QuotedPrefix returns the quoted string (as understood by Unquote) at the prefix of s. If s does not start with a valid quoted string, QuotedPrefix returns an error.
func Unquote ¶
Unquote interprets s as a single-quoted, double-quoted, or backquoted Go string literal, returning the string value that s quotes. (If s is single-quoted, it would be a Go character literal; Unquote returns the corresponding one-character string.)
func UnquoteChar ¶
UnquoteChar decodes the first character or byte in the escaped string or character literal represented by the string s. It returns four values:
- value, the decoded Unicode code point or byte value;
- multibyte, a boolean indicating whether the decoded character requires a multibyte UTF-8 representation;
- tail, the remainder of the string after the character; and
- an error that will be nil if the character is syntactically valid.
The second argument, quote, specifies the type of literal being parsed and therefore which escaped quote character is permitted. If set to a single quote, it permits the sequence \' and disallows unescaped '. If set to a double quote, it permits \" and disallows unescaped ". If set to zero, it does not permit either escape and allows both quote characters to appear unescaped.
type NumError ¶
A NumError records a failed conversion.
func (*NumError) Error ¶
Func (*numerror) unwrap ¶ added in go1.14, source files ¶.
- eisel_lemire.go
Keyboard shortcuts
: This menu | |
: Search site | |
or | : Jump to |
or | : Canonical URL |
Why can you ignore a returned error without an underscore?
Perhaps there is a simple reason for this… but I don’t understand why the compiler allows this:
It’s not that hard to forget that the hello function returns an error (in a more complicated scenario) and if you were just code reviewing main (assume the hello func is in some lib) a reviewer would definitely miss the fact that an error might have occurred.
Running example: The Go Playground
If I saw a call to a function that took no params and returned nothing, I would probably examine it. I don’t think the Go compiler is trying to prevent you from doing certain things like ignoring errors. It’s just trying to make you do so intentionally. This, to me, seems intentional.
I’ve been bitten by plenty of things while writing software. Can’t say that calling a function named hello while ignoring that it might return something has been one of them. Especially in go, given that it’s idiomatic to return errors if errors are possible. And thus it’s idiomatic to also check for errors. What’s the real-world scenario you are trying to protect against?
I guess my example was quite contrived. I was just trying to illustrate the function signature that would cause the issue I was asking about. The context for the question is that I have been working to bring Go into my team at work - so I’ve been trying to learn a lot about the smaller edge cases in the language and to understand where a new Go programmer might make mistakes.
I would also counter that Go does attempt to ensure you don’t make simple mistakes (maybe not errors specifically since they are just another value). To me, my hello example would be the same thing as attempting to do this (again just a contrived example for illustrative purposes):
Which of course does not compile. Instead, the compiler says: ./prog.go:12:11: assignment mismatch: 1 variable but executeUpdate returns 2 values .
Why doesn’t my hello example fail to compile with the same type of error (e.g. assignment mismatch: 0 variables but hello returns 1 value )?
I would expect that you would need to write _ = hello() for the compiler to accept it (which is OK as well… but not required).
I should note that I don’t think anyone disagrees that this is bad code and not idiomatic Go. I’m just trying to understand why the compiler doesn’t enforce it because it seems like a simple case that would ensure cleaner and easier to read code. I also know (don’t have an exact specific example) that I’ve copy/pasted function signatures and forgot to handle the error and when the compiler doesn’t complain, I forget. So it’s not about trying to take a short cut so much as trying to catch simple mistakes.
Here’s a GitHub issue with some discussion:
Some creative solutions were put forward but it didn’t get much traction and at this point it would break the compatibility promise .
Ah! Thank you for sharing that. I missed that in my search. I think that pretty much answers my question then - and makes sense that at this point it would break backwards compatibility.
They make a reference to this package: GitHub - kisielk/errcheck: errcheck checks that you checked errors.
Which I think would probably satisfy my concern in terms of catching mistakes. Perhaps a bit noisy, but it looks like you can supply an allow-list for functions that you don’t care about.
I’ll give that a try and keep track of those proposals to see where they land. Thanks again!
This topic was automatically closed 90 days after the last reply. New replies are no longer allowed.
Package strconv
Package strconv implements conversions to and from string representations of basic data types.
Numeric Conversions
The most common numeric conversions are Atoi (string to int) and Itoa (int to string).
These assume decimal and the Go int type.
ParseBool, ParseFloat, ParseInt, and ParseUint convert strings to values:
The parse functions return the widest type (float64, int64, and uint64), but if the size argument specifies a narrower width the result can be converted to that narrower type without data loss:
FormatBool, FormatFloat, FormatInt, and FormatUint convert values to strings:
AppendBool, AppendFloat, AppendInt, and AppendUint are similar but append the formatted value to a destination slice.
String Conversions
Quote and QuoteToASCII convert strings to quoted Go string literals. The latter guarantees that the result is an ASCII string, by escaping any non-ASCII Unicode with \u:
QuoteRune and QuoteRuneToASCII are similar but accept runes and return quoted Go rune literals.
Unquote and UnquoteChar unquote Go string and rune literals.
Package files
atob.go atof.go atoi.go decimal.go doc.go extfloat.go ftoa.go isprint.go itoa.go quote.go
Internal call graph ▹
Internal call graph ▾.
In the call graph viewer below, each node is a function belonging to this package and its children are the functions it calls—perhaps dynamically.
The root nodes are the entry points of the package: functions that may be called from outside the package. There may be non-exported or anonymous functions among them if they are called dynamically from another package.
Click a node to visit that function's source code. From there you can visit its callers by clicking its declaring func token.
Functions may be omitted if they were determined to be unreachable in the particular programs or tests that were analyzed.
IntSize is the size in bits of an int or uint value.
ErrRange indicates that a value is out of range for the target type.
ErrSyntax indicates that a value does not have the right syntax for the target type.

func AppendBool ¶
AppendBool appends "true" or "false", according to the value of b, to dst and returns the extended buffer.
func AppendFloat ¶
AppendFloat appends the string form of the floating-point number f, as generated by FormatFloat, to dst and returns the extended buffer.
func AppendInt ¶
AppendInt appends the string form of the integer i, as generated by FormatInt, to dst and returns the extended buffer.
func AppendQuote ¶
AppendQuote appends a double-quoted Go string literal representing s, as generated by Quote, to dst and returns the extended buffer.
func AppendQuoteRune ¶
AppendQuoteRune appends a single-quoted Go character literal representing the rune, as generated by QuoteRune, to dst and returns the extended buffer.
func AppendQuoteRuneToASCII ¶
AppendQuoteRuneToASCII appends a single-quoted Go character literal representing the rune, as generated by QuoteRuneToASCII, to dst and returns the extended buffer.
func AppendQuoteRuneToGraphic ¶
AppendQuoteRuneToGraphic appends a single-quoted Go character literal representing the rune, as generated by QuoteRuneToGraphic, to dst and returns the extended buffer.
func AppendQuoteToASCII ¶
AppendQuoteToASCII appends a double-quoted Go string literal representing s, as generated by QuoteToASCII, to dst and returns the extended buffer.
func AppendQuoteToGraphic ¶
AppendQuoteToGraphic appends a double-quoted Go string literal representing s, as generated by QuoteToGraphic, to dst and returns the extended buffer.
func AppendUint ¶
AppendUint appends the string form of the unsigned integer i, as generated by FormatUint, to dst and returns the extended buffer.
func Atoi ¶
Atoi returns the result of ParseInt(s, 10, 0) converted to type int.
func CanBackquote ¶
CanBackquote reports whether the string s can be represented unchanged as a single-line backquoted string without control characters other than tab.
func FormatBool ¶
FormatBool returns "true" or "false" according to the value of b
func FormatFloat ¶
FormatFloat converts the floating-point number f to a string, according to the format fmt and precision prec. It rounds the result assuming that the original was obtained from a floating-point value of bitSize bits (32 for float32, 64 for float64).
The format fmt is one of 'b' (-ddddp±ddd, a binary exponent), 'e' (-d.dddde±dd, a decimal exponent), 'E' (-d.ddddE±dd, a decimal exponent), 'f' (-ddd.dddd, no exponent), 'g' ('e' for large exponents, 'f' otherwise), or 'G' ('E' for large exponents, 'f' otherwise).
The precision prec controls the number of digits (excluding the exponent) printed by the 'e', 'E', 'f', 'g', and 'G' formats. For 'e', 'E', and 'f' it is the number of digits after the decimal point. For 'g' and 'G' it is the total number of digits. The special precision -1 uses the smallest number of digits necessary such that ParseFloat will return f exactly.
func FormatInt ¶
FormatInt returns the string representation of i in the given base, for 2 <= base <= 36. The result uses the lower-case letters 'a' to 'z' for digit values >= 10.
func FormatUint ¶
FormatUint returns the string representation of i in the given base, for 2 <= base <= 36. The result uses the lower-case letters 'a' to 'z' for digit values >= 10.
func IsGraphic ¶
IsGraphic reports whether the rune is defined as a Graphic by Unicode. Such characters include letters, marks, numbers, punctuation, symbols, and spaces, from categories L, M, N, P, S, and Zs.
func IsPrint ¶
IsPrint reports whether the rune is defined as printable by Go, with the same definition as unicode.IsPrint: letters, numbers, punctuation, symbols and ASCII space.
func Itoa ¶
Itoa is shorthand for FormatInt(int64(i), 10).
func ParseBool ¶
ParseBool returns the boolean value represented by the string. It accepts 1, t, T, TRUE, true, True, 0, f, F, FALSE, false, False. Any other value returns an error.
func ParseFloat ¶
ParseFloat converts the string s to a floating-point number with the precision specified by bitSize: 32 for float32, or 64 for float64. When bitSize=32, the result still has type float64, but it will be convertible to float32 without changing its value.
If s is well-formed and near a valid floating point number, ParseFloat returns the nearest floating point number rounded using IEEE754 unbiased rounding.
The errors that ParseFloat returns have concrete type *NumError and include err.Num = s.
If s is not syntactically well-formed, ParseFloat returns err.Err = ErrSyntax.
If s is syntactically well-formed but is more than 1/2 ULP away from the largest floating point number of the given size, ParseFloat returns f = ±Inf, err.Err = ErrRange.
func ParseInt ¶
ParseInt interprets a string s in the given base (2 to 36) and returns the corresponding value i. If base == 0, the base is implied by the string's prefix: base 16 for "0x", base 8 for "0", and base 10 otherwise.
The bitSize argument specifies the integer type that the result must fit into. Bit sizes 0, 8, 16, 32, and 64 correspond to int, int8, int16, int32, and int64.
The errors that ParseInt returns have concrete type *NumError and include err.Num = s. If s is empty or contains invalid digits, err.Err = ErrSyntax and the returned value is 0; if the value corresponding to s cannot be represented by a signed integer of the given size, err.Err = ErrRange and the returned value is the maximum magnitude integer of the appropriate bitSize and sign.
func ParseUint ¶
ParseUint is like ParseInt but for unsigned numbers.
func Quote ¶
Quote returns a double-quoted Go string literal representing s. The returned string uses Go escape sequences (\t, \n, \xFF, \u0100) for control characters and non-printable characters as defined by IsPrint.
func QuoteRune ¶
QuoteRune returns a single-quoted Go character literal representing the rune. The returned string uses Go escape sequences (\t, \n, \xFF, \u0100) for control characters and non-printable characters as defined by IsPrint.
func QuoteRuneToASCII ¶
QuoteRuneToASCII returns a single-quoted Go character literal representing the rune. The returned string uses Go escape sequences (\t, \n, \xFF, \u0100) for non-ASCII characters and non-printable characters as defined by IsPrint.
func QuoteRuneToGraphic ¶
QuoteRuneToGraphic returns a single-quoted Go character literal representing the rune. The returned string uses Go escape sequences (\t, \n, \xFF, \u0100) for non-ASCII characters and non-printable characters as defined by IsGraphic.
func QuoteToASCII ¶
QuoteToASCII returns a double-quoted Go string literal representing s. The returned string uses Go escape sequences (\t, \n, \xFF, \u0100) for non-ASCII characters and non-printable characters as defined by IsPrint.
func QuoteToGraphic ¶
QuoteToGraphic returns a double-quoted Go string literal representing s. The returned string uses Go escape sequences (\t, \n, \xFF, \u0100) for non-ASCII characters and non-printable characters as defined by IsGraphic.
func Unquote ¶
Unquote interprets s as a single-quoted, double-quoted, or backquoted Go string literal, returning the string value that s quotes. (If s is single-quoted, it would be a Go character literal; Unquote returns the corresponding one-character string.)
func UnquoteChar ¶
UnquoteChar decodes the first character or byte in the escaped string or character literal represented by the string s. It returns four values:
The second argument, quote, specifies the type of literal being parsed and therefore which escaped quote character is permitted. If set to a single quote, it permits the sequence \' and disallows unescaped '. If set to a double quote, it permits \" and disallows unescaped ". If set to zero, it does not permit either escape and allows both quote characters to appear unescaped.
type NumError ¶
A NumError records a failed conversion.
func (*NumError) Error ¶
Converting string to integer with error handling - strconv, Itoa
- Table of Contents (t)
- Indexed keywords (k)
- Chapter TOC (d)
- Hide/Show (h)
- Copyright 2024 Gábor Szabó
- Last updated at 2024-06-30 09:30:47.382428
Get the Reddit app
Ask questions and post articles about the Go programming language and related tools, events etc.
assignment mismatch error
I have a simple sqlite query...
func main() {
database, _ := sql.Open ("sqlite3", "./database.db")
select_user, _ := database.Prepare("SELECT username FROM users WHERE username='ANDY'")
query := select_user.Exec()
fmt.Println("The SELECT statement returned "+ query)
But I get this error...
assignment mismatch: 1 variable but select_user.Exec returns 2 values
However we can see from the client it returns a single string...
sqlite> SELECT username FROM users WHERE username='ANDY';
So why does this not work ? Thanks !!
StrConv Function
Returns a Variant ( String ) converted as specified.
StrConv ( string, conversion [ , LCID ] )
The StrConv function syntax has these arguments:
|
|
| Required. String expression to be converted. |
| Required. Integer. The sum of values specifying the type of conversion to perform. |
| Optional. The LocaleID, if different than the system LocaleID. (The system LocaleID is the default.) |
The conversion argument settings are:
|
|
|
| 1 | Converts the string to uppercase characters. |
| 2 | Converts the string to lowercase characters. |
| 3 | Converts the first letter of every word in string to uppercase. |
| 4* | Converts narrow (single-byte) characters in string to wide (double-byte) characters. |
| 8* | Converts wide (double-byte) characters in string to narrow (single-byte) characters. |
| 16** | Converts Hiragana characters in string to Katakana characters. |
| 32** | Converts Katakana characters in string to Hiragana characters. |
| 64 | Converts the string to Unicode using the default code page of the system. (Not available on the Macintosh.) |
| 128 | Converts the string from Unicode to the default code page of the system. (Not available on the Macintosh.) |
*Applies to East Asia locales.
**Applies to Japan only.
Note: These constants are specified by Visual Basic for Applications (VBA). As a result, they may be used anywhere in your code in place of the actual values. Most can be combined, for example, vbUpperCase + vbWide , except when they are mutually exclusive, for example, vbUnicod e + vbFromUnicode . The constants vbWide , vbNarrow , vbKatakana , and vbHiragana cause run-time errors when used in locales where they do not apply.
The following are valid word separators for proper casing: Null ( Chr $( 0 ) ), horizontal tab ( Chr $( 9 ) ), linefeed ( Chr $( 10 ) ), vertical tab ( Chr $( 11 ) ), form feed ( Chr $( 12 ) ), carriage return ( Chr $( 13 ) ), space (SBCS) ( Chr $( 32 ) ). The actual value for a space varies by country/region for DBCS.
When you're converting from a Byte array in ANSI format to a string, you should use the StrConv function. When you're converting from such an array in Unicode format, use an assignment statement.
Query examples
|
|
SELECT strConv(ProductDesc,1) AS Expr1 FROM ProductSales; | Converts the values from “ProductDesc” field into uppercase and displays in column Expr1 |
SELECT strConv(ProductDesc,2) AS LowercaseID FROM ProductSales; | Converts the values from “ProductDesc” field into lowercase and displays in column LowercaseID. Converts the first letter of every word from "ProductDesc" to uppercase and displays in column PropercaseID. All other characters are left as lowercase. |
VBA example
Note: Examples that follow demonstrate the use of this function in a Visual Basic for Applications (VBA) module. For more information about working with VBA, select Developer Reference in the drop-down list next to Search and enter one or more terms in the search box.
This example uses the StrConv function to convert a Unicode string to an ANSI string.
String functions and how to use them

Need more help?
Want more options.
Explore subscription benefits, browse training courses, learn how to secure your device, and more.
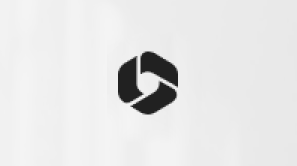
Microsoft 365 subscription benefits
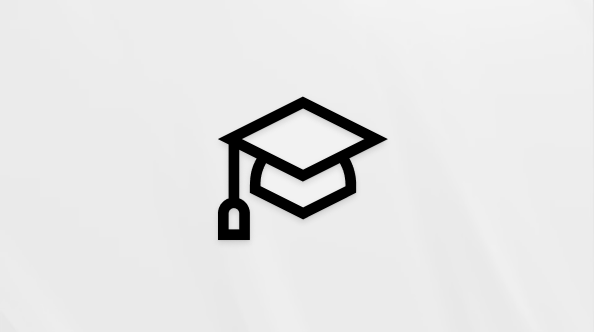
Microsoft 365 training
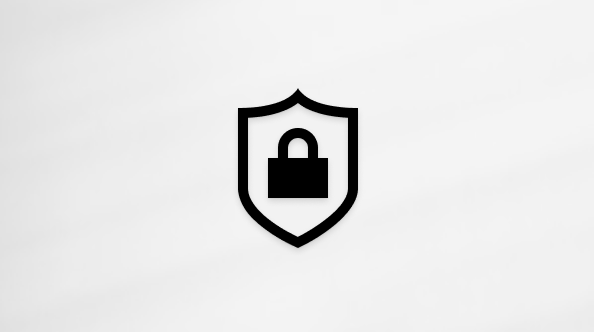
Microsoft security
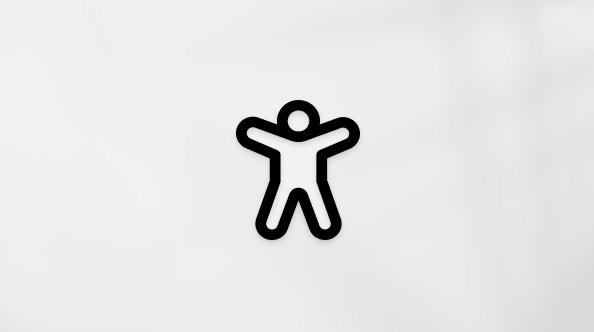
Accessibility center
Communities help you ask and answer questions, give feedback, and hear from experts with rich knowledge.

Ask the Microsoft Community
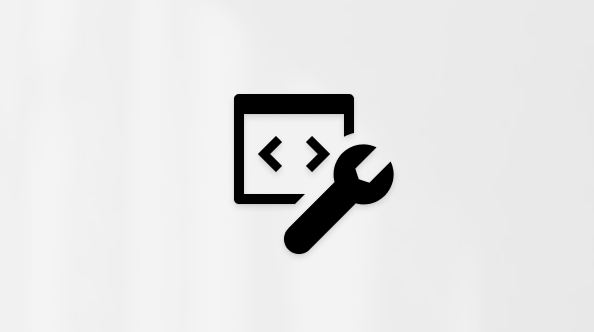
Microsoft Tech Community
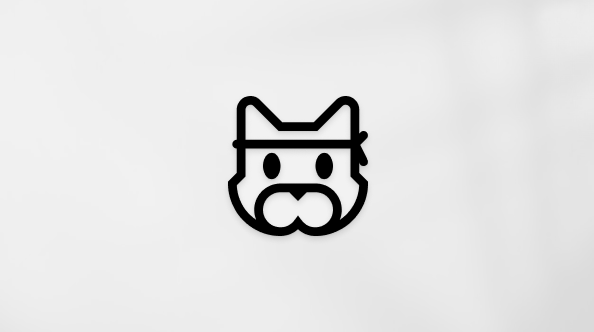
Windows Insiders
Microsoft 365 Insiders
Was this information helpful?
Thank you for your feedback.
- เข้าสู่ระบบ
- สมัครสมาชิก
assignment mismatch: 1 variable but strconv.Atoi returns 2 values Go คือ วิธีแก้ไข
รันโค้ด Go (Golang) รับค่าจากผู้ใช้งานและแปลงเป็นตัวเลข แต่พอรันแล้วขึ้น assignment mismatch: 1 variable but strconv.Atoi returns 2 values ต้องแก้ไขอย่างไร
ปัญหานี้เกิดจากคำสั่ง strconv.Atoi (แปลง String เป็น Integer) จะคืนค่า (Return) มา 2 ค่า คือค่าที่แปลง และค่ากรณีเกิด error ให้แก้ไขตรงส่วนตัวแปร number := เป็น number, _ := ความหมายของตัวขีดล่าง (_) คือให้โค้ดดังกล่าวไม่สนใจ error ( skip error) สามารถเขียนโปรแกรมได้ดังนี้
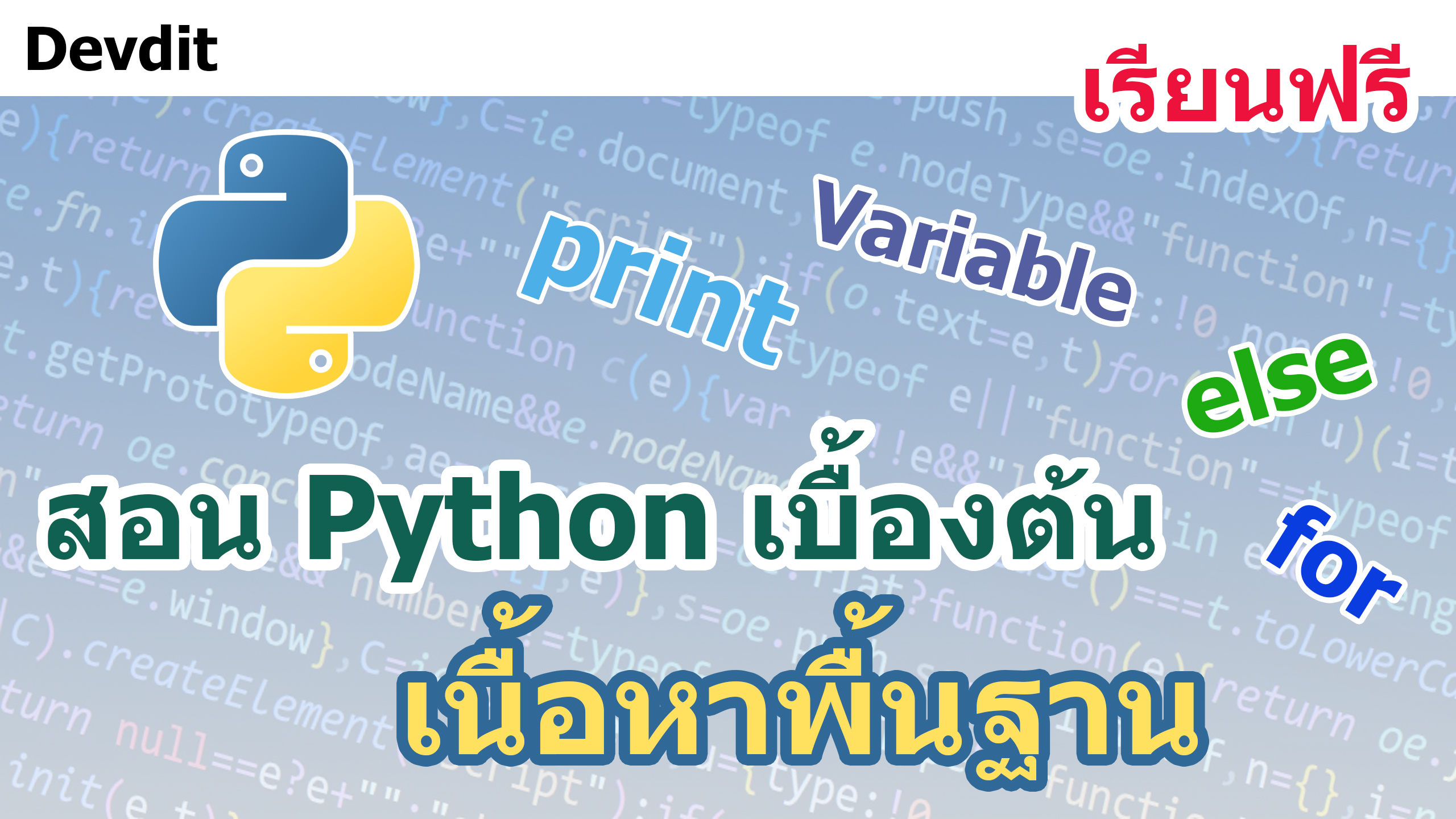
- Python เพิ่มค่าลง List แบบหลายค่า ด้วย extend และการบวก
- JavaScript น้อยกว่า น้อยกว่าหรือเท่ากับ
- Python แสดงข้อมูลจาก 2 ตารางแบบ join ตาราง
- ภาษา C เปลี่ยน พ.ศ. เป็น ค.ศ.
- โค้ดเลือกสี HTML ตารางสี
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Golang syntax error : assignment mismatch: 4 variables but 2 values [closed]
The code below produces a compilation error.
After grappling with this error for hours, I still can't figure out what to do with this.
Here is an simplified example which produces the same error:
Obviously, k,ok1,h,ok2 := a(),b(); leads to this error. But I don't know how to modify this code.
For some reason, I can't mov this assignment statement out of if-else block. Is there any way I can fix this problem?
- syntax-error
- variable-assignment

- 1 If that would be allowed it would be dangerous as it could lead to subtle bugs. – Volker Commented Mar 24, 2020 at 15:02
This is the relevant section in the language spec:
https://golang.org/ref/spec#Assignments
is invalid go syntax. The "...right hand operand is a single multi-valued expression...". Above, you have two expressions. You have to do the assignment separately:
And the if statement becomes:
If you don't want those variables outside that scope, do this:

Not the answer you're looking for? Browse other questions tagged go syntax-error variable-assignment or ask your own question .
- Featured on Meta
- Upcoming sign-up experiments related to tags
- Policy: Generative AI (e.g., ChatGPT) is banned
- The [lib] tag is being burninated
- What makes a homepage useful for logged-in users
Hot Network Questions
- Why was the animal "Wolf" used in the title "The Wolf of Wall Street (2013)"?
- Folk stories and notions in mathematics that are likely false, inaccurate, apocryphal, or poorly founded?
- Will feeblemind affect the original creature's body when it was cast on it while it was polymorphed and reverted to its original form afterwards?
- Do known physical systems all have a unique time evolution?
- Viewport Shader Render different from 1 computer to another
- Can I tell a MILP solver to prefer solutions with fewer fractions?
- Why can't LaTeX (seem to?) Support Arbitrary Text Sizes?
- Why is the forward process referred to as the "ground truth" in diffusion models?
- Have children's car seats not been proven to be more effective than seat belts alone for kids older than 24 months?
- How to "refresh" or "reset" the settings of a default view?
- How do you say "living being" in Classical Latin?
- Does "*some of the town council*" mean the same as "*some of the **members** of the town council*"?
- Next date in the future such that all 8 digits of MM/DD/YYYY are all different and the product of MM, DD and YY is equal to YYYY
- Correlation for Small Dataset?
- How can I confirm for sure that a CVE has been mitigated on a RHEL system?
- Visit USA via land border for French citizen
- Is there an image viewer for ubuntu that will read/apply xmp sidecars?
- Are there any CID episodes based on real-life events?
- Would the category of directed sets be better behaved with the empty set included, or excluded?
- Is it unfair to retroactively excuse a student for absences?
- Have there been any scholarly attempts and/or consensus as regards the missing lines of "The Ruin"?
- Can I route audio from a macOS Safari PWA to specific speakers, different from my system default?
- Sets of algebraic integers whose differences are units
- How do guitarists remember what note each string represents when fretting?
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
assignment mismatch: 2 variables but thrift.NewTSocketConf returns 1 value #52
Lay523 commented Oct 12, 2022
version:v0.13.0
|
The text was updated successfully, but these errors were encountered: |
citrusreticulata commented Oct 17, 2022
Please check the version of thrift and the version of . In thrift 0.15.0, function returns 1 value. It's specific implementation of this function is as follows: |
Sorry, something went wrong.
For more details of differences between two version, please take a look at this PR: |
citrusreticulata commented Feb 16, 2023
Has this issue been solved? If this issue has been solved, it can be closed. |
No branches or pull requests

IMAGES
VIDEO
COMMENTS
When trying to use strconv on a variable passed via URL(GET variable named times), GoLang fails on compilation stating the following: multiple-value strconv.Atoi() in a single-value context. However, when I do reflect.TypeOf I get string as the type, which to my understanding is the correct type of argument.
This function is returning 2 values, thus we have to use 2 variables. So you have to use. config := mollie.NewConfig(true, mollie.OrgTokenEnv) mollieClient, err := mollie.NewClient(client, config) assignment mismatch: 1 variable but mollie.NewClient returns 2 values will be resolved with this change.
This package provides an Atoi () function which is equivalent to ParseInt (str string, base int, bitSize int) is used to convert string type into int type. To access Atoi () function you need to import strconv Package in your program. Syntax: func Atoi(str string) (int, error) Here, str is the string. Example 1:
The most common numeric conversions are Atoi (string to int) and Itoa (int to string). i, err := strconv.Atoi("-42") s := strconv.Itoa(-42) These assume decimal and the Go int type. ParseBool, ParseFloat, ParseInt, and ParseUint convert strings to values: b, err := strconv.ParseBool("true")
Which of course does not compile. Instead, the compiler says: ./prog.go:12:11: assignment mismatch: 1 variable but executeUpdate returns 2 values. ... (e.g. assignment mismatch: 0 variables but hello ... // Should be t = t.Add(…) ``` ```go strconv.AppendQuote(dst, "suffix") // Should be dst = strconv.AppendQuote(…) ``` For the few cases ...
assignment mismatch: 2 variables but 1 values #4. Closed amirkheirabadi73 opened this issue Mar 11, 2018 · 3 comments Closed assignment mismatch: 2 variables but 1 values #4. amirkheirabadi73 opened this issue Mar 11, 2018 · 3 comments Comments. Copy link
The most common numeric conversions are Atoi (string to int) and Itoa (int to string). i, err := strconv.Atoi ("-42") s := strconv.Itoa (-42) These assume decimal and the Go int type. ParseBool, ParseFloat, ParseInt, and ParseUint convert strings to values:
42 int strconv.Atoi: parsing "23\n": invalid syntax strconv.Atoi: parsing "17x": invalid syntax This can, of course go wrong. If we ask for an integer and the user types in "42x" or even "FooBar". So the conversion might fail. ... the actual value we are interested in and another value that we assign to a variable called err.
$ go run main.go # dns-cli/cmd cmd/subdomainDelete.go:38:6: undefined: recordID cmd/subdomainDelete.go:41:9: assignment mismatch: 2 variables but 1 values cmd/subdomainDelete.go:41:40: undefined: recordID I've only been using golang for about six months and this is my first ever project using cobra. So far, I have two other commands working ...
assignment mismatch: 2 variables but uuid.NewV4 returns 1 values #106. ... Open assignment mismatch: 2 variables but uuid.NewV4 returns 1 values #106. barry-en opened this issue Mar 5, 2020 · 6 comments Comments. Copy link barry-en commented Mar 5, 2020. I use go mod go mod init
assignment mismatch: 1 variable but select_user.Exec returns 2 values However we can see from the client it returns a single string... sqlite> SELECT username FROM users WHERE username='ANDY';
Saved searches Use saved searches to filter your results more quickly
StrConv ( string, conversion [, LCID ] ) The StrConv function syntax has these arguments: Required. String expression to be converted. Required. Integer. The sum of values specifying the type of conversion to perform. Optional. The LocaleID, if different than the system LocaleID.
assignment mismatch: 2 variables but uuid.NewV4 returns 1 values #34280. Closed chingiz2387 opened this issue Sep 13, 2019 · 3 comments Closed assignment mismatch: 2 variables but uuid.NewV4 returns 1 values #34280. chingiz2387 opened this issue Sep 13, 2019 · 3 ... "cannot initialize 2 variables with 1 values", "source": "LSP ...
number := strconv.Atoi( scanner.Text() ) number *= 2. fmt.Println( number ) } วิธีแก้ไข. ปัญหานี้เกิดจากคำสั่ง strconv.Atoi (แปลง String เป็น Integer) จะคืนค่า (Return) มา 2 ค่า คือค่าที่แปลง และค่า ...
1 Answer Sorted by: Reset to default Highest score (default) Trending (recent votes count more) Date modified (newest first) Date created (oldest first)
In thrift 0.14.1, function NewTSocketConf returns 2 values. It's specific implementation of this function is as follows: It's specific implementation of this function is as follows: func NewTSocketConf(hostPort string, conf *TConfiguration) (*TSocket, error) { addr, err := net.ResolveTCPAddr("tcp", hostPort) if err != nil { return nil, err ...